This primitive executes a Lua script, either a system one or a custom one.
There is no SimPe wizard for this instruction, so the following table details the meaning of each of the operands.
Operand | Description | Operand Values |
---|---|---|
0 & 1 | The STR# resource to locate the script in. Usually set to 0x0130. | |
2 & 3 | One more than the index of the entry to use from the STR# given in operands 0 and 1. So to use entry 0x00 set this value to 0x0001. | |
4 - bit 1 | Controls where the script is | Off: Custom code is in the description field of the STR# entry On: System script (code is pre-compiled as a .objLua resource). |
4 - bit 2 | Controls if the STR# resource is private | Off: If bit 3 is also off, scope is global On: Scope is private |
4 - bit 3 | Controls if the STR# resource is semi-global | Off: If bit 2 is also off, scope is global On: Scope is semi-global |
4 - bit 4 | Controls if arguments are used | Off: No args, data is extracted from SO and Temps On: Args are in operands 6 thru 14 |
4 - bits 5 thru 8 | Unused | |
5 | Unused. | |
6 | Parameter 0 (if Op4 bit4 is 1), can be retrieve from the Lua script as GetPrimitiveParameter(0) | |
7 & 8 | Qualifiers on the variable in operand 6 | |
9 | Parameter 1 (if Op4 bit4 is 1), can be retrieve from the Lua script as GetPrimitiveParameter(1) | |
10 & 11 | Qualifiers on the variable in operand 9 | |
12 | Parameter 2 (if Op4 bit4 is 1), can be retrieve from the Lua script as GetPrimitiveParameter(2) | |
13 & 14 | Qualifiers on the variable in operand 12 | |
15 | Unused |
GetObjectTile
Gets the tile the object is located on; (0, 0, 0) is ground level, top right of lot.
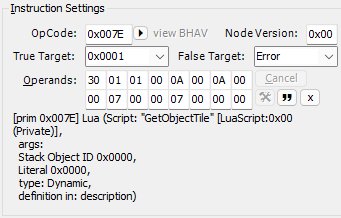
-- Tile x/y/z values are integers (so you can't tell if an object is on quarter tile placement) local m_oid = GetPrimitiveParameter(0) local m_tileX, m_tileY, m_tileZ = nWorld.GetObjectTile(m_oid) if (m_tileX ~= nil) then SetTemp(0, m_tileX) SetTemp(1, m_tileY) SetTemp(2, m_tileZ) SetScriptReturnValue(true) else SetScriptReturnValue(false) end return
SetObjectTile
Move the object to the centre of the specified tile; (0, 0, 0) is ground level, top right of lot.
local m_oid = GetPrimitiveParameter(0) local m_tileX = GetTemp(0); local m_tileY = GetTemp(1); local m_tileZ = GetTemp(2) if (nWorld.MoveObjectToLocation(m_oid, m_tileX + 0.5, m_tileY + 0.5, m_tileZ)) then SetScriptReturnValue(true) else SetScriptReturnValue(false) end return
GetObjectPosition
Gets the position of the object; (0, 0, 0) is ground level, top right of lot.
-- Position x/y are floats to 2dp; position z is an integer local m_oid = GetPrimitiveParameter(0) local m_posX, m_posY, m_posZ = nWorld.GetObjectLocation(m_oid) if (m_posX ~= nil) then SetTemp(0, m_posX * 100) SetTemp(1, m_posY * 100) SetTemp(2, m_posZ) SetScriptReturnValue(true) else SetScriptReturnValue(false) end return
SetObjectPosition
Move the object to the given position, position x and y are "times 100", so 5.25 is given as 525
local m_oid = GetPrimitiveParameter(0) local m_posX = GetTemp(0) / 100; local m_posY = GetTemp(1) / 100; local m_posZ = GetTemp(2) if (nWorld.MoveObjectToLocation(m_oid, m_posX, m_posY, m_posZ)) then SetScriptReturnValue(true) else SetScriptReturnValue(false) end return
SetObjectTileRandom
Moves the object to the centre of a random tile, which may be on the road/pavement.
local m_oid = GetPrimitiveParameter(0) local m_sizeX = nWorld.GetLotSizeX() local m_sizeY = nWorld.GetLotSizeY() local m_maxTries = 25 local m_tileZ = 0 while (m_maxTries > 0) do m_maxTries = m_maxTries - 1 local m_tileX = math.random(m_sizeX) local m_tileY = math.random(m_sizeY) if (nWorld.MoveObjectToLocation(m_oid, m_tileX + 0.5, m_tileY + 0.5, m_tileZ)) then SetScriptReturnValue(true) return end end SetScriptReturnValue(false) return
SetObjectPositionRandom
Moves the object to a random position, which may be on the road/pavement.
local m_oid = GetPrimitiveParameter(0) local m_sizeX = nWorld.GetLotSizeX() local m_sizeY = nWorld.GetLotSizeY() local m_maxTries = 25 local m_posZ = 0 while (m_maxTries > 0) do m_maxTries = m_maxTries - 1 local m_posX = math.random(m_sizeX) + (math.random(3) / 4.0) local m_posY = math.random(m_sizeY) + (math.random(3) / 4.0) if (nWorld.MoveObjectToLocation(m_oid, m_posX, m_posY, m_posZ)) then SetScriptReturnValue(true) return end end SetScriptReturnValue(false) return
- Dizzy's Lua Notes @MTS
- AdidasSG2's Lua Tutorial (Part 6) @MTS
- tunaisafish's decompiled game scripts @MATY
// Decompiled with JetBrains decompiler // Type: pjse.BhavNameWizards.WizPrim0x007e // Assembly: pjse.coder.plugin, Version=4.0.3349.37576, Culture=neutral, PublicKeyToken=null using SimPe.PackedFiles.Wrapper; using System; namespace pjse.BhavNameWizards { public class WizPrim0x007e : BhavWizPrim { public WizPrim0x007e(Instruction i) : base(i) { } protected override string Operands(bool lng) { byte[] numArray = new byte[16]; ((byte[]) this.instruction.Operands).CopyTo((Array) numArray, 0); ((byte[]) this.instruction.Reserved1).CopyTo((Array) numArray, 8); ushort num = BhavWiz.ToShort(numArray[4], numArray[5]); string str1 = ""; if (lng) str1 = str1 + Localization.GetString("bwp7e_script") + ": "; string str2; if (BhavWiz.ToShort(numArray[2], numArray[3]) != (ushort) 0) { Scope scope = Scope.Global; if (((int) num & 2) != 0) scope = Scope.Private; else if (((int) num & 4) != 0) scope = Scope.SemiGlobal; str2 = str1 + this.readStr(scope, (uint) BhavWiz.ToShort(numArray[0], numArray[1]), (ushort) ((uint) BhavWiz.ToShort(numArray[2], numArray[3]) - 1U), lng ? -1 : 60, lng ? Detail.Full : Detail.Errors, false); if (((int) num & 8) != 0) { str2 += lng ? ", " + Localization.GetString("manyArgs") + ": " : ", "; for (int index = 0; index < 3; ++index) str2 = str2 + (index != 0 ? ", " : "") + this.dataOwner(lng, numArray[6 + 3 * index], numArray[7 + 3 * index], numArray[8 + 3 * index]); } if (lng) str2 = str2 + ", " + Localization.GetString("bwp7e_type") + ": " + (((int) num & 1) != 0 ? Localization.GetString("bwp7e_definition") : Localization.GetString("bwp7e_dynamic")) + ", " + Localization.GetString("bwp7e_definitionIn") + ": " + (((int) num & 1) != 0 ? Localization.GetString("bwp7e_objLuaFile") : Localization.GetString("bwp7e_description")); } else str2 = str1 + Localization.GetString("none"); return str2; } } }